These functions enable you to access Apache internal features-they form a high-level interface to some Apache API functions. Consequently, these functions are available only if you have compiled PHP as an Apache module. It's important to remember that these functions are case-sensitive. Functions such as apache_note() make a distinction between uppercase and lowercase variables, just like normal PHP variables.
Opens an Apache subrequest to look up status information for a URI (Uniform Resource Identifier).
Returns:
Class containing a list of status information for a URI
Description:
If you have installed PHP as an Apache module, you can use this function to get information about a URI. Just as with virtual() or an SSI-include, the function opens an Apache subrequest; therefore, you can specify only a local document URI as the parameter.
The following properties will be set in the returned class:
allowed
content_type
args
filename
boundary
handler
byterange
method
bytes_sent
mtime
clength
no_cache
no_local_copy
status_line
path_info
the_request
request_time
unparsed_uri
status
uri
Version:
Existing since version 3.0.4
Example:
Get the system filename of the top-level index.html file
$uri = apache_lookup_uri("/index.html");
print("filename: ". $uri->filename);
$arr = get_object_vars($uri);
while (list($prop, $val) = each($arr)) {
echo "<BR /> $prop = $val \n";
}
apache_note
mixed apache_note(string key, [string value])
key
Name of the note entry
value
Value of the note entry
Gets or sets Apache note entries.
Returns:
Value of the Apache note corresponding to the key specified as the first argument; FALSE if no entry was found for the specified key
Description:
Apache maintains an internal notes table-a simple list of key/value pairs-for every request; with this function, you can get and set entries from this table. The notes table will be flushed as soon as the request has been processed.
To set a new notes entry, specify its name as the first argument and its value as the second. If you leave out the second argument, the function returns the contents of the notes entry corresponding to the non-case-sensitive key specified as the first argument. If no matching entry is found, the function returns FALSE. Note that if you set an entry, the function returns the value of the old entry, if one was found, or FALSE otherwise.
apache_note() is helpful in passing information between modules that operate at different stages of the request. For example, it could be used for logging: You could create an Apache note entry listing the currently logged-in user and include the username in the server logs using mod_log_config with a custom directive such as "%{username}n".
Version:
Existing since version 3.0.2
Warning:
In versions prior to 3.0.3, apache_note() corrupted memory and produced segfaults in some circumstances.
Example:
Set an Apache notes entry listing the currently logged-in user
apache_note("username", $PHP_REMOTE_USER);
ascii2ebcdic
int ascii2ebcdic(string ascii)
Converts ASCII text to EBCDIC.
Returns:
Converted text
Description:
Converts an ASCII encoded string to its EBCDIC equivalent. EBCDIC is a binary-safe encoding and is available only on EBCDIC-enabled operating systems such as AS/400 and BS2000/OSD.
Version:
Existing since version 3.0.17
See also:
ebcdic2ascii
Example:
Convert an ASCII string to EBCDIC
echo ascii2ebcdic("Hello World");
ebcdic2ascii
int ebcdic2ascii(string ebcdic)
Converts an EBCDIC string to ASCII.
Returns:
Converted text
Description:
Converts an EBCDIC-encoded string to its ASCII equivalent. EBCDIC is a binary-safe encoding and is only available on EBCDIC-enabled operating systems such as AS/400 and BS2000/OSD.
Version:
Existing since version 3.0.17
See also:
ascii2ebcdic
Example:
Convert an EBCDIC string to ASCII
echo ebcdic2ascii("Hello World");
getallheaders
array getallheaders()
Gets the HTTP headers that the client sent.
Returns:
Associative array containing a list of HTTP headers, or FALSE on error
Description:
Call this function to retrieve an array of all HTTP headers sent by the client browser to the server. This function returns an associative array filled with the headers, in which each key is the name of the header, and each data element is the value associated with that name. If you have safe_mode enabled in PHP, the "authorization" header isn't included in the result set.
Header names are not case-sensitive. A client may send "User-Agent: foo" or "user-agent: foo", or use any other combination of upper- and lowercase for the header name. However, the name of the key in the associative array returned by this function will exactly match the case of the name sent by the client, so looking for the "User-Agent" key in the array will not return the expected value if the client used "user-agent" as the header name.
Warning:
In versions prior to 3.0.6, problems occurred when key/value pairs were empty.
Version:
Existing since version 3.0
Example:
Output the client's User-Agent HTTP header
$headers = getallheaders();
if ($headers) {
$useragent = $headers["User-Agent"];
print("You are using $useragent.
\n");
}
virtual
int virtual(string URI)
Performs an Apache subrequest to parse and include a file.
Returns:
TRUE if successful; FALSE on error
Description:
This function enables you to perform an Apache subrequest. It performs the same function that mod_include allows you to perform (), but you can access the feature from within PHP scripts. This is useful if you want to include the output of a CGI script within your PHP file, for example. You can't use virtual() on PHP files; use include() instead.
Version:
Existing since version 3.0
Warning:
In versions prior to 3.0.13, it was possible to use virtual() on PHP files under some circumstances.
Example:
Include the output of a Perl CGI script
$script = "/cgi-bin/script.pl";
if (!@virtual($script)) {
// The @ prevents a PHP error from occurring if the function call fails
print("ERROR - Unable to include file '$script'!
\n");
}
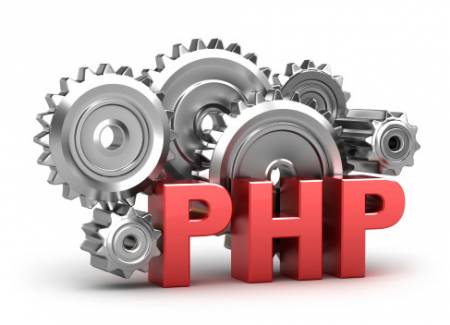
Code
apache_lookup_uri
class apache_lookup_uri(string URI)
class apache_lookup_uri(string URI)
Opens an Apache subrequest to look up status information for a URI (Uniform Resource Identifier).
Returns:
Class containing a list of status information for a URI
Description:
If you have installed PHP as an Apache module, you can use this function to get information about a URI. Just as with virtual() or an SSI-include, the function opens an Apache subrequest; therefore, you can specify only a local document URI as the parameter.
The following properties will be set in the returned class:
allowed
content_type
args
filename
boundary
handler
byterange
method
bytes_sent
mtime
clength
no_cache
no_local_copy
status_line
path_info
the_request
request_time
unparsed_uri
status
uri
Version:
Existing since version 3.0.4
Example:
Get the system filename of the top-level index.html file
Code
$uri = apache_lookup_uri("/index.html");
print("filename: ". $uri->filename);
$arr = get_object_vars($uri);
while (list($prop, $val) = each($arr)) {
echo "<BR /> $prop = $val \n";
}
apache_note
mixed apache_note(string key, [string value])
key
Name of the note entry
value
Value of the note entry
Gets or sets Apache note entries.
Returns:
Value of the Apache note corresponding to the key specified as the first argument; FALSE if no entry was found for the specified key
Description:
Apache maintains an internal notes table-a simple list of key/value pairs-for every request; with this function, you can get and set entries from this table. The notes table will be flushed as soon as the request has been processed.
To set a new notes entry, specify its name as the first argument and its value as the second. If you leave out the second argument, the function returns the contents of the notes entry corresponding to the non-case-sensitive key specified as the first argument. If no matching entry is found, the function returns FALSE. Note that if you set an entry, the function returns the value of the old entry, if one was found, or FALSE otherwise.
apache_note() is helpful in passing information between modules that operate at different stages of the request. For example, it could be used for logging: You could create an Apache note entry listing the currently logged-in user and include the username in the server logs using mod_log_config with a custom directive such as "%{username}n".
Version:
Existing since version 3.0.2
Warning:
In versions prior to 3.0.3, apache_note() corrupted memory and produced segfaults in some circumstances.
Example:
Set an Apache notes entry listing the currently logged-in user
Code
apache_note("username", $PHP_REMOTE_USER);
ascii2ebcdic
int ascii2ebcdic(string ascii)
Converts ASCII text to EBCDIC.
Returns:
Converted text
Description:
Converts an ASCII encoded string to its EBCDIC equivalent. EBCDIC is a binary-safe encoding and is available only on EBCDIC-enabled operating systems such as AS/400 and BS2000/OSD.
Version:
Existing since version 3.0.17
See also:
ebcdic2ascii
Example:
Convert an ASCII string to EBCDIC
Code
echo ascii2ebcdic("Hello World");
ebcdic2ascii
int ebcdic2ascii(string ebcdic)
Converts an EBCDIC string to ASCII.
Returns:
Converted text
Description:
Converts an EBCDIC-encoded string to its ASCII equivalent. EBCDIC is a binary-safe encoding and is only available on EBCDIC-enabled operating systems such as AS/400 and BS2000/OSD.
Version:
Existing since version 3.0.17
See also:
ascii2ebcdic
Example:
Convert an EBCDIC string to ASCII
Code
echo ebcdic2ascii("Hello World");
getallheaders
array getallheaders()
Gets the HTTP headers that the client sent.
Returns:
Associative array containing a list of HTTP headers, or FALSE on error
Description:
Call this function to retrieve an array of all HTTP headers sent by the client browser to the server. This function returns an associative array filled with the headers, in which each key is the name of the header, and each data element is the value associated with that name. If you have safe_mode enabled in PHP, the "authorization" header isn't included in the result set.
Header names are not case-sensitive. A client may send "User-Agent: foo" or "user-agent: foo", or use any other combination of upper- and lowercase for the header name. However, the name of the key in the associative array returned by this function will exactly match the case of the name sent by the client, so looking for the "User-Agent" key in the array will not return the expected value if the client used "user-agent" as the header name.
Warning:
In versions prior to 3.0.6, problems occurred when key/value pairs were empty.
Version:
Existing since version 3.0
Example:
Output the client's User-Agent HTTP header
Code
$headers = getallheaders();
if ($headers) {
$useragent = $headers["User-Agent"];
print("You are using $useragent.
\n");
}
virtual
Code
int virtual(string URI)
Performs an Apache subrequest to parse and include a file.
Returns:
TRUE if successful; FALSE on error
Description:
This function enables you to perform an Apache subrequest. It performs the same function that mod_include allows you to perform (), but you can access the feature from within PHP scripts. This is useful if you want to include the output of a CGI script within your PHP file, for example. You can't use virtual() on PHP files; use include() instead.
Version:
Existing since version 3.0
Warning:
In versions prior to 3.0.13, it was possible to use virtual() on PHP files under some circumstances.
Example:
Include the output of a Perl CGI script
Code
$script = "/cgi-bin/script.pl";
if (!@virtual($script)) {
// The @ prevents a PHP error from occurring if the function call fails
print("ERROR - Unable to include file '$script'!
\n");
}